Password Protected File Validation for(.doc/.docx/.xls/.xlsx/.pdf) file types
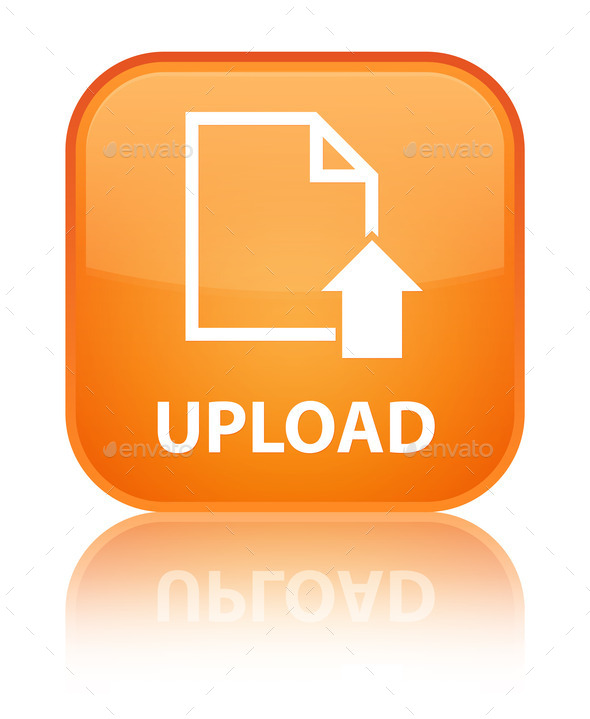
protected void btnUpload_Click(object sender, EventArgs e)
{
//Check
if File Upload control has file or not
if (FileUpload1.HasFile)
{
//Get
Uploaded file bytes
var bytes = FileUpload1.FileBytes;
//Get
Uploaded File Extension
FileInfo objFileInfo = new FileInfo(FileUpload1.FileName);
string StrFileExt = objFileInfo.Extension.ToUpper();
//Based
on the File extension call appropriate user defined method.
//For
PDF file type
if (StrFileExt == ".PDF")
{
//Upload and save file in server temp folder
var newfilename = DateTime.Now.GetHashCode() +
FileUpload1.FileName;
FileUpload1.SaveAs(System.Web.Hosting.HostingEnvironment.MapPath("~/UploadedFiles/" + newfilename));
//Validate Password Protected PDF.
if (IsPasswordProtectedPDF(newfilename))
{
ShowMsg(true);
}
else
{
ShowMsg(false);
}
}
//For
Word and Excel file types
else if ((StrFileExt == ".DOC") || (StrFileExt == ".DOCX") || (StrFileExt == ".XLS") || (StrFileExt == ".XLSX"))
{
if (IsPassworded(bytes))
{
ShowMsg(true);
}
else
{
ShowMsg(false);
}
}
//Show
msg on invalid file upload
else
{
lblmsg.Text = "Invalid file format!!!";
lblmsg.ForeColor =
System.Drawing.Color.Red;
}
}
}
public static bool IsPasswordProtectedPDF(string strNewFileName)
{
try
{
PdfDocument document = PdfReader.Open(System.Web.Hosting.HostingEnvironment.MapPath("~/UploadedFiles/" + strNewFileName), "1234");
return false;
}
catch (PdfReaderException)
{
return true;
}
}
public void ShowMsg(bool status)
{
if (status)
{
lblmsg.Text = "Yes, It's password protected!!";
lblmsg.ForeColor =
System.Drawing.Color.Red;
}
else
{
lblmsg.Text = "No, It's not password protected!!";
lblmsg.ForeColor =
System.Drawing.Color.Green;
}
}
public static bool IsPassworded(byte[] bytes)
{
var prefix = Encoding.Default.GetString(bytes.Take(2).ToArray());
if (prefix == "ÐÏ")
{
//Office
format.
//Flagged
with password
if (bytes.Skip(0x20c).Take(1).ToArray()[0] == 0x2f) return true; //XLS 2003
if (bytes.Skip(0x214).Take(1).ToArray()[0] == 0x2f) return true; //XLS 2005
if (bytes.Skip(0x20B).Take(1).ToArray()[0] == 0x13) return true; //DOC 2005
if (bytes.Length < 2000) return false; //Guessing false
var start = Encoding.Default.GetString(bytes.Take(2000).ToArray()); //DOC/XLS 2007+
start = start.Replace("\0", " ");
if (start.Contains("E
n c r y p t e d P a c k a g e")) return true;
return false;
}
//Unknown.
return false;
}
protected void btnUpload_Click(object sender, EventArgs e)
Comments
Post a Comment